Receiving a payment
Here you can find how to receive a payment in a few steps
Creating a payment link
To create a Payment Link you can do a POST
request to /payments/requests:
{
"amount": 10,
"description": "My payment request"
}
You will get the following response:
{
"id": "bb236f8d-caa5-47eb-97bf-688475037f3e",
"amount": 10,
"description": "My payment request",
"status": "CREATED",
"createdAt": "2023-11-14T16:57:17.511Z",
"updatedAt": "2023-11-14T16:57:17.511Z",
"callbackUrls": null,
"paymentUrl": "https://pay.pluggy.ai/bb236f8d-caa5-47eb-97bf-688475037f3e"
}
Now you can send the paymentUrl
to the person you want to pay they can open it in any browser and complete the payment
Feature only by invite
For the moment, this feature is in beta and has to be enabled for your client specifically.
If you're interested in trying it out, please contact our Sales team!
PIX QR Payments
You can also create a payment request that completes payment to a PIX QR code, doing a POST
to the /payments/requests/pix-qr endpoint:
{
"pixQrCode": "00020126490014br.gov.bcb.pix0108dict-key0215additional-info52040000530398654031005802BR5912example-name6006Cidade62090505tx-id63045E20"
}
In pixQrCode
you send the text contained within the PIX QR image. Using Payment Initiation enhances PIX QR codes allowing you to be notified when the payment was completed (via callbackUrls
or webhooks)
Payment flow
When the user enters the payment URL, they will enter the following flow:
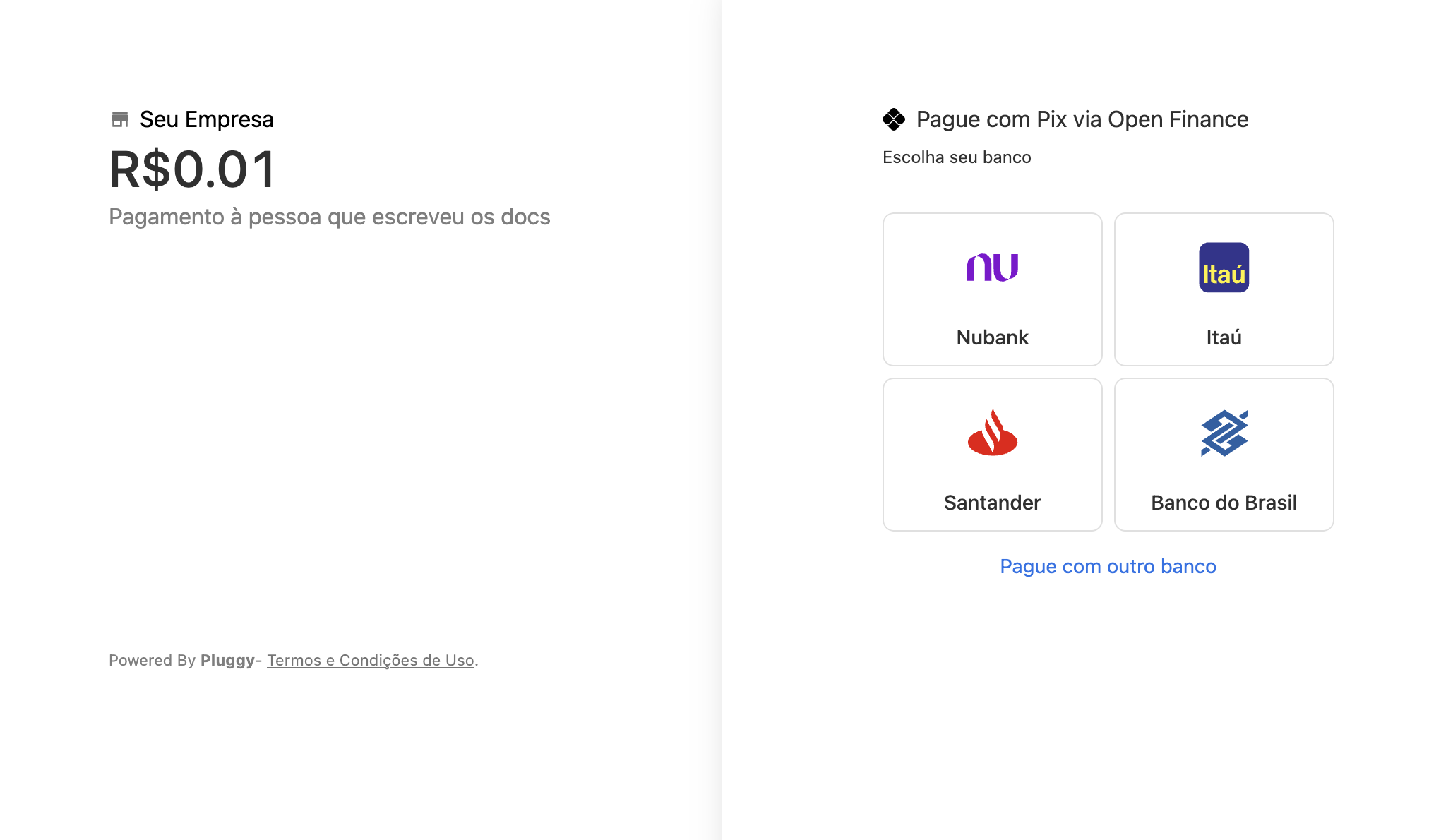
First they choose the institution to do the payment
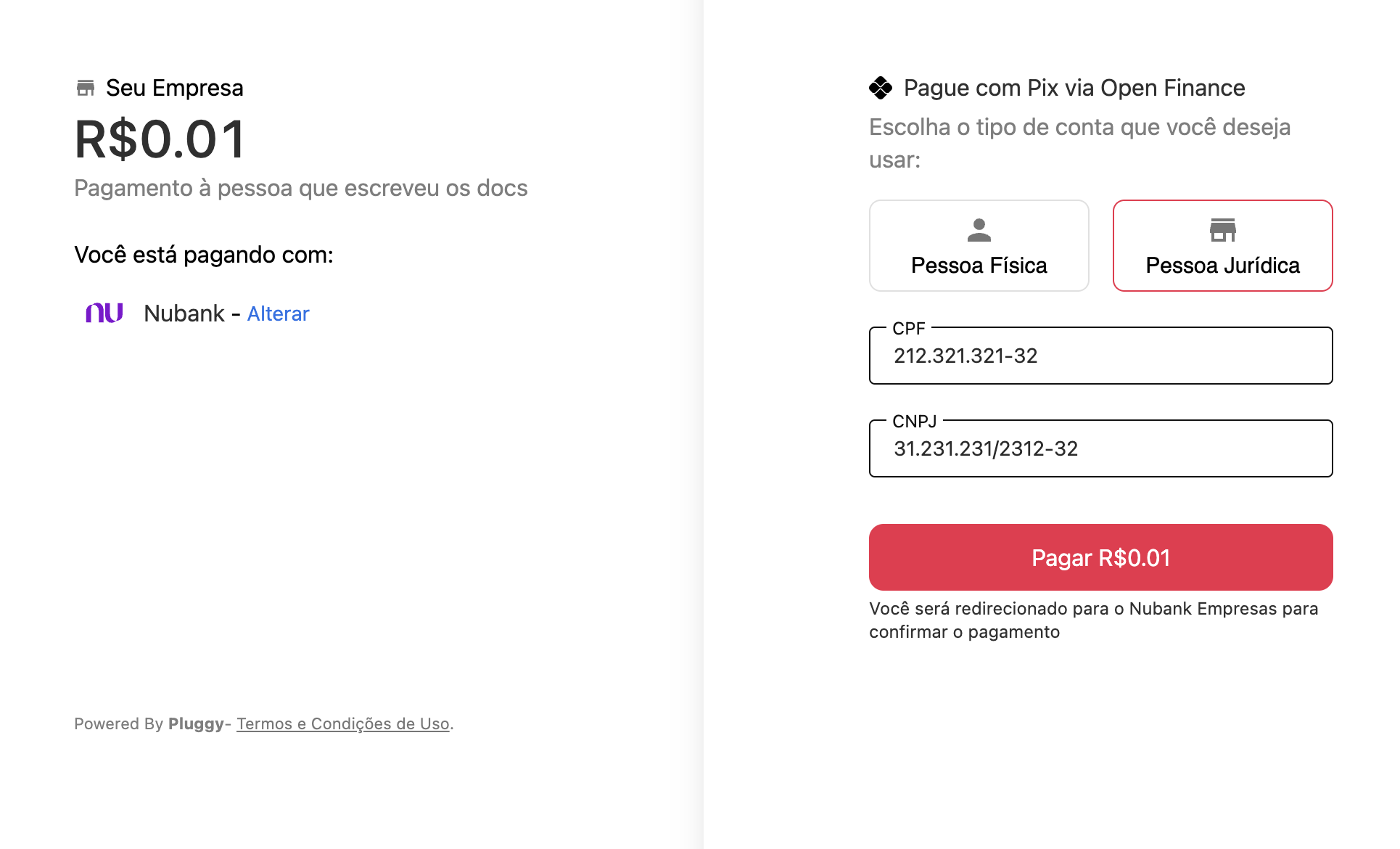
Confirm details, input CPF/CNPJ and authorize payment within the institution's app/webiste
After the User completes the flow, they will see a Success or Failure screen
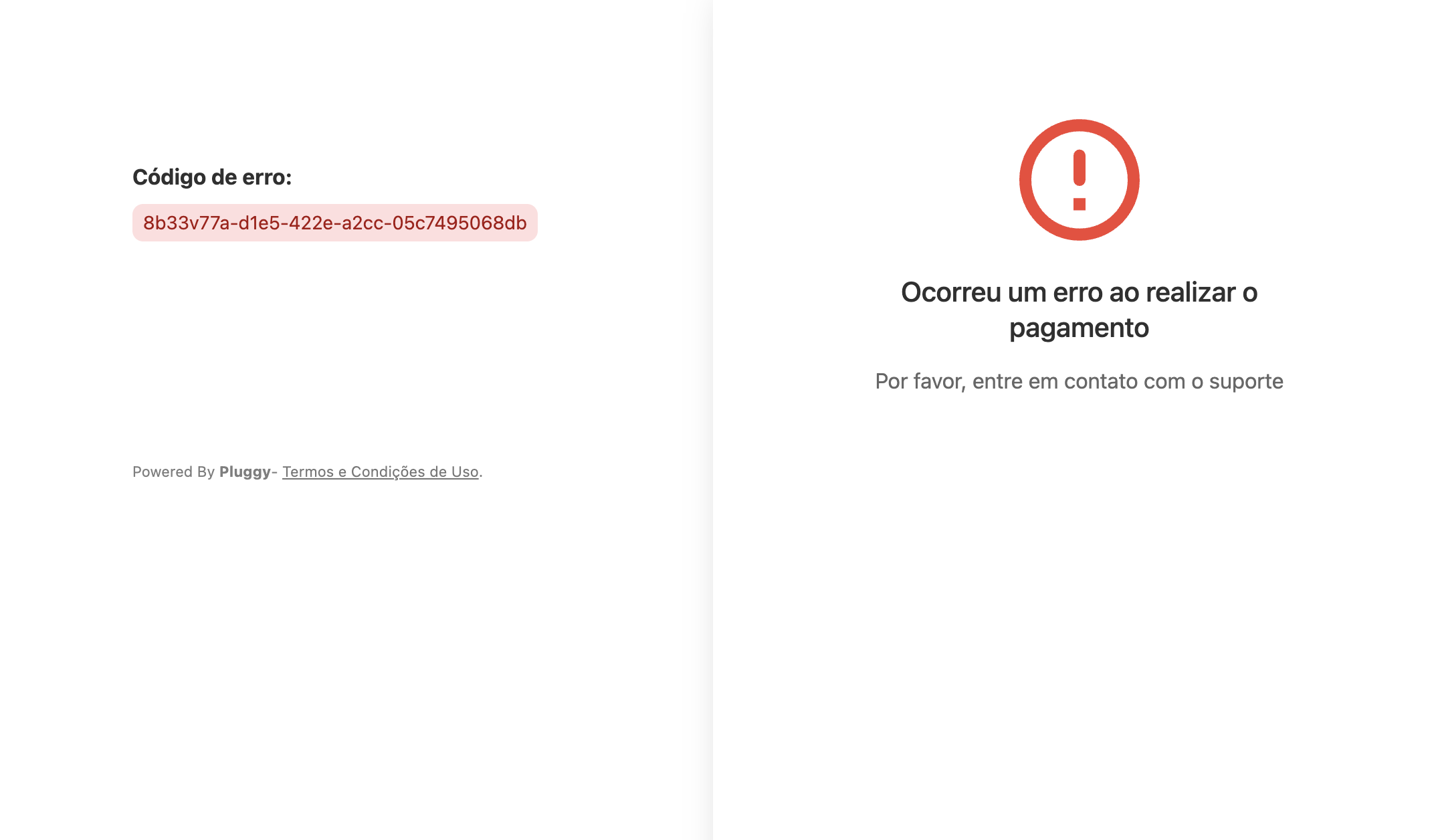
Error screen
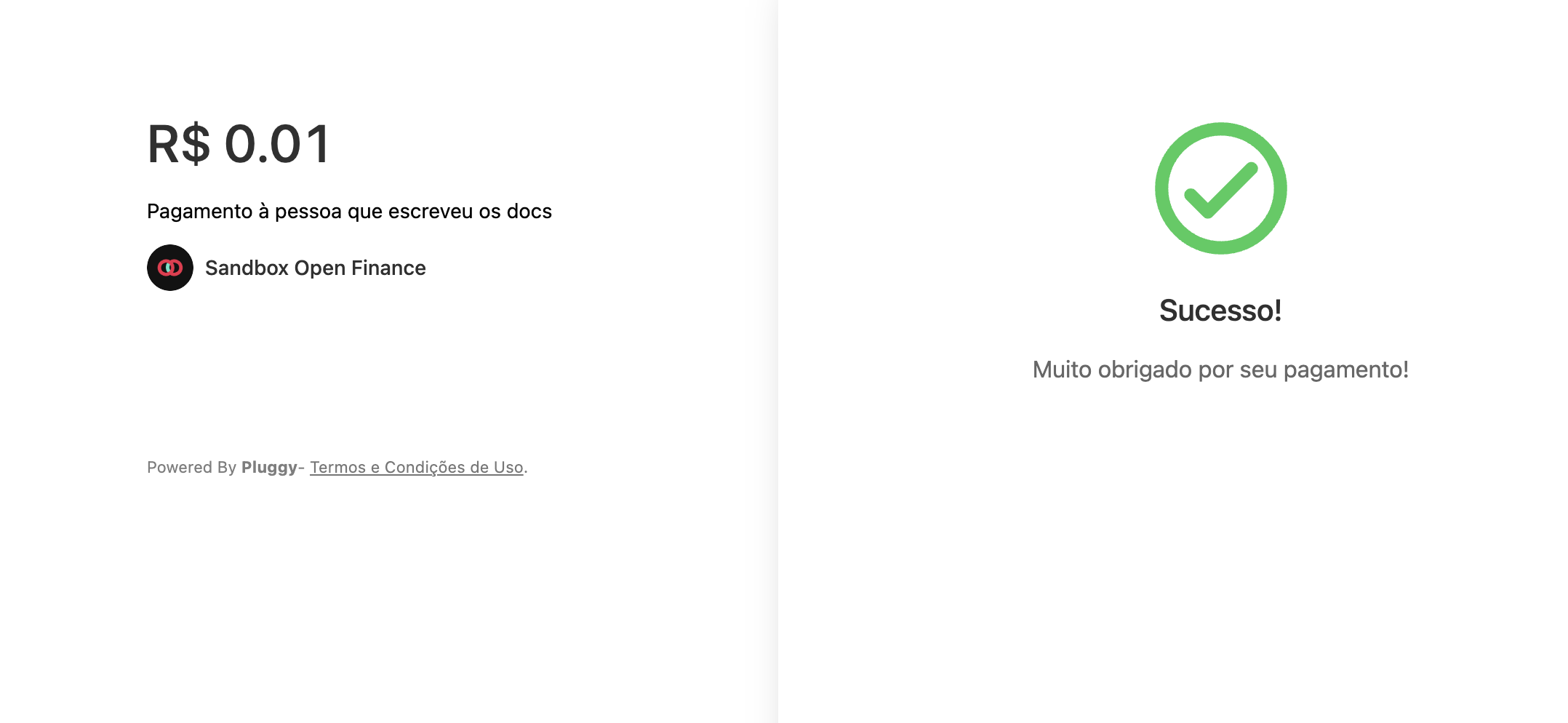
Success screen
Custom Result screen (callbacks)
You can use your custom Result screens by specifying callbackUrls in Payment Creation, see docs here
Creating a custom payment experience
If you don't want to use the default pay.pluggy.ai
flow, you can implement your custom payment experience by following this:
First of all, you need to create a Payment Request. There, you will specify how much money you want to receive. Also, you can configure a description (to be shown to the final user at the moment they authorize the payment), and a set of callback URLs where the user will be redirected after the payment authorization was completed. Both description
and callbackUrl
fields are optional.
{
"amount": 100.50,
"description": "Transferência",
"callbackUrls": {
"success": "https://my-success-url.com",
"error": "https://my-error-url.com",
"pending": "https://my-pending-url.com"
}
}
{
"id": "05c693bf-c196-47ea-a28c-8251d6bb8a06",
"amount": 100.50,
"description": "Transferência",
"status": "CREATED",
"createdAt": "2023-11-06T13:03:45.689Z",
"updatedAt": "2023-11-06T13:03:45.689Z",
"callbackUrls": {
"success": "https://my-success-url.com",
"error": "https://my-error-url.com"
}
}
You can find the endpoint details here
Creating a Payment Intent
After creating a payment request, you need to create a Payment Intent. This represents an intent of a person to make that payment. For example, if you want to charge a customer R$10, first you need to create a Payment Request for that amount, and then a Payment Intent when the user wants to pay.
To create a Payment Intent, you need to send the ID of the institution (connectorId
) that the user will use to make the payment. You can find the connector list using our connector's endpoint and filter the ones with supportsPaymentInitiation
property with value true
. Also, you need to send the institution's required credentials in the parameters
field. Those credentials also can be found in the connector's endpoint.
This is the request to create a Payment Intent.
{
"paymentRequestId": "05c693bf-c196-47ea-a28c-8251d6bb8a06",
"connectorId": 601,
"parameters": {
"cpf": "76109277673"
}
}
{
"paymentRequestId": "05c693bf-c196-47ea-a28c-8251d6bb8a06",
"connectorId": 618,
"parameters": {
"cpf": "76109277673",
"cnpj": "11111111111111"
}
}
{
"id": "4316602b-8fb5-4bfd-92dc-32921b7414f1",
"status": "CONSENT_AWAITING_AUTHORIZATION",
"createdAt": "2023-11-09T20:10:42.706Z",
"updatedAt": "2023-11-09T20:10:42.706Z",
"paymentRequest": {
"id": "f6696d02-3583-47ae-b195-148d71b8ae9b",
"amount": 100.50,
"description": null,
"status": "IN_PROGRESS",
"createdAt": "2023-11-09T20:10:25.084Z",
"updatedAt": "2023-11-09T20:10:42.706Z",
"callbackUrls": {
"success": "https://my-success-url.com",
"error": "https://my-error-url.com"
}
},
"connector": {
"id": 601,
"name": "Itaú",
"primaryColor": "48be9d",
"institutionUrl": "https://cdn.raidiam.io/directory-ui/brand/obbrazil/0.2.0.112/favicon.svg",
"country": "BR",
"type": "PERSONAL_BANK",
"credentials": [
{
"validation": "^\\d{3}\\.?\\d{3}\\.?\\d{3}-?\\d{2}$",
"validationMessage": "CPF deve ter 11 números.",
"label": "CPF",
"name": "cpf",
"type": "number",
"placeholder": "",
"optional": false
}
],
"imageUrl": "https://cdn.pluggy.ai/assets/connector-icons/itau.svg",
"hasMFA": false,
"oauth": true,
"health": {
"status": "ONLINE",
"stage": null
},
"products": [
"ACCOUNTS",
"TRANSACTIONS",
"IDENTITY",
"CREDIT_CARDS",
"PAYMENT_DATA",
"LOANS",
"INVESTMENTS"
],
"createdAt": "2023-07-24T14:29:32.140Z",
"isSandbox": true,
"isOpenFinance": true,
"updatedAt": "2023-11-09T19:17:08.495Z",
"supportsPaymentInitiation": true
},
"consentUrl": "https://consent-url.com"
}
There are important properties in the response object:
- status
At this point, it will haveCONSENT_AWAITING_AUTHORIZATION
as value. It means the user needs to authorize it in the institution. You can check the other possible values here - consentUrl
It is the URL where you need to redirect the user in order to authorize the payment (it expires after 5 minutes). After the payment is completed, the user will be redirected to the URLs specified in thecallbackUrls
defined before, or to a default URL provided by Pluggy if it wasn't specified.
You can find the endpoint details here
Testing payments with Sandbox bank
You can use our testing app (playground)[https://playground.pluggy.ai]
to test the flow without any setup!
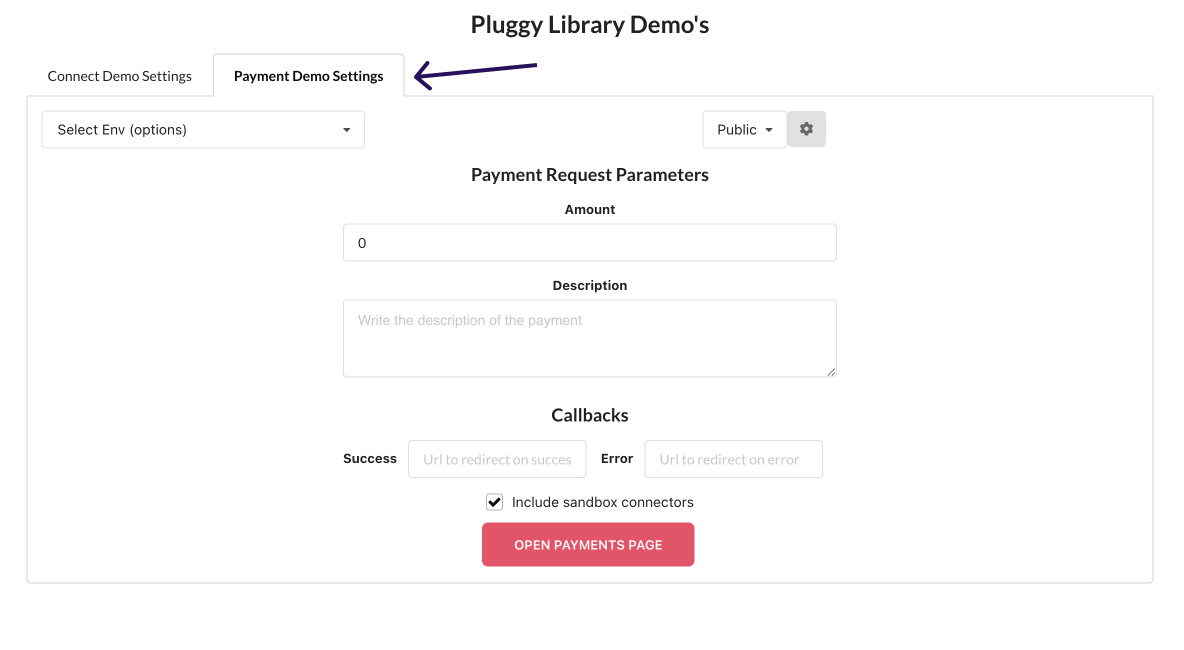
Using sandbox without playground
To use Sandbox you in our App
https://pay.pluggy.ai/
you need to specify the query param
?include_sandbox=true
.
After config the app with your clientId
and clientSecret
You can Open the payments page and use Sandbox connector with the following credentials:
{
cpf: "76109277673",
user: "[email protected]",
password: "P@ssword01"
}
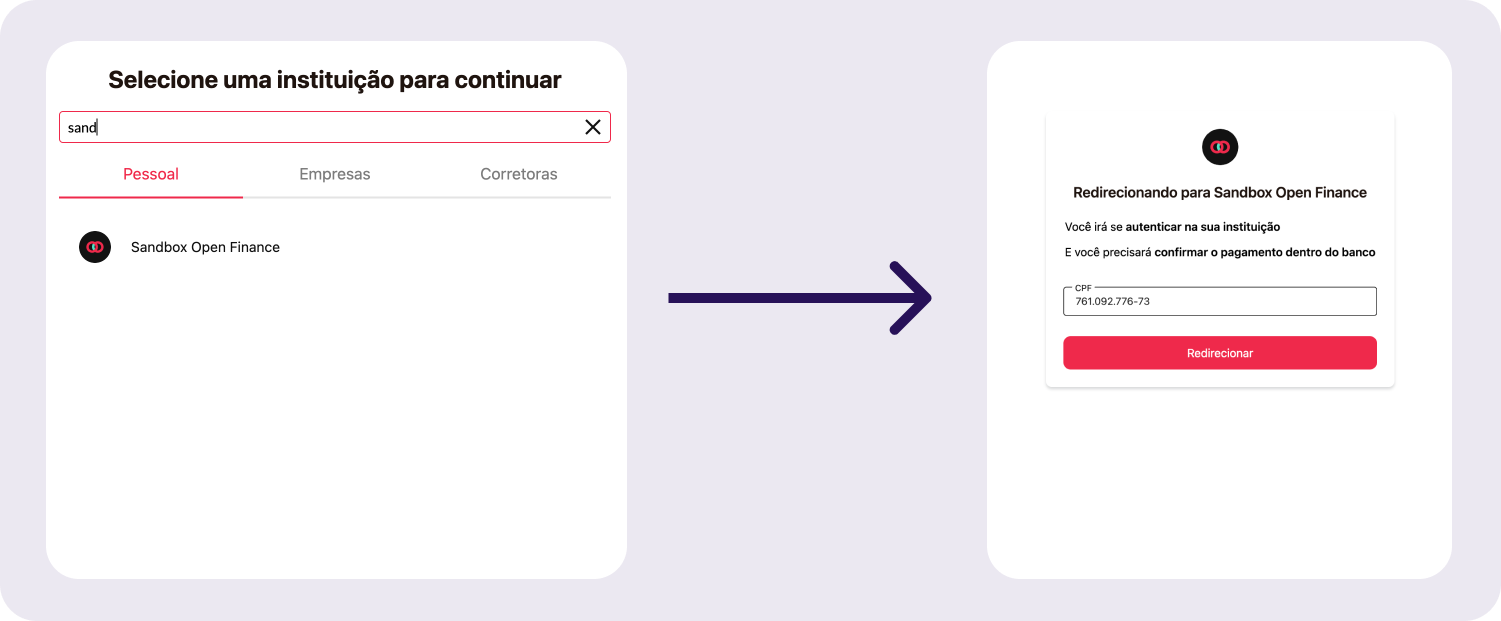
Use CPF credential in first Input
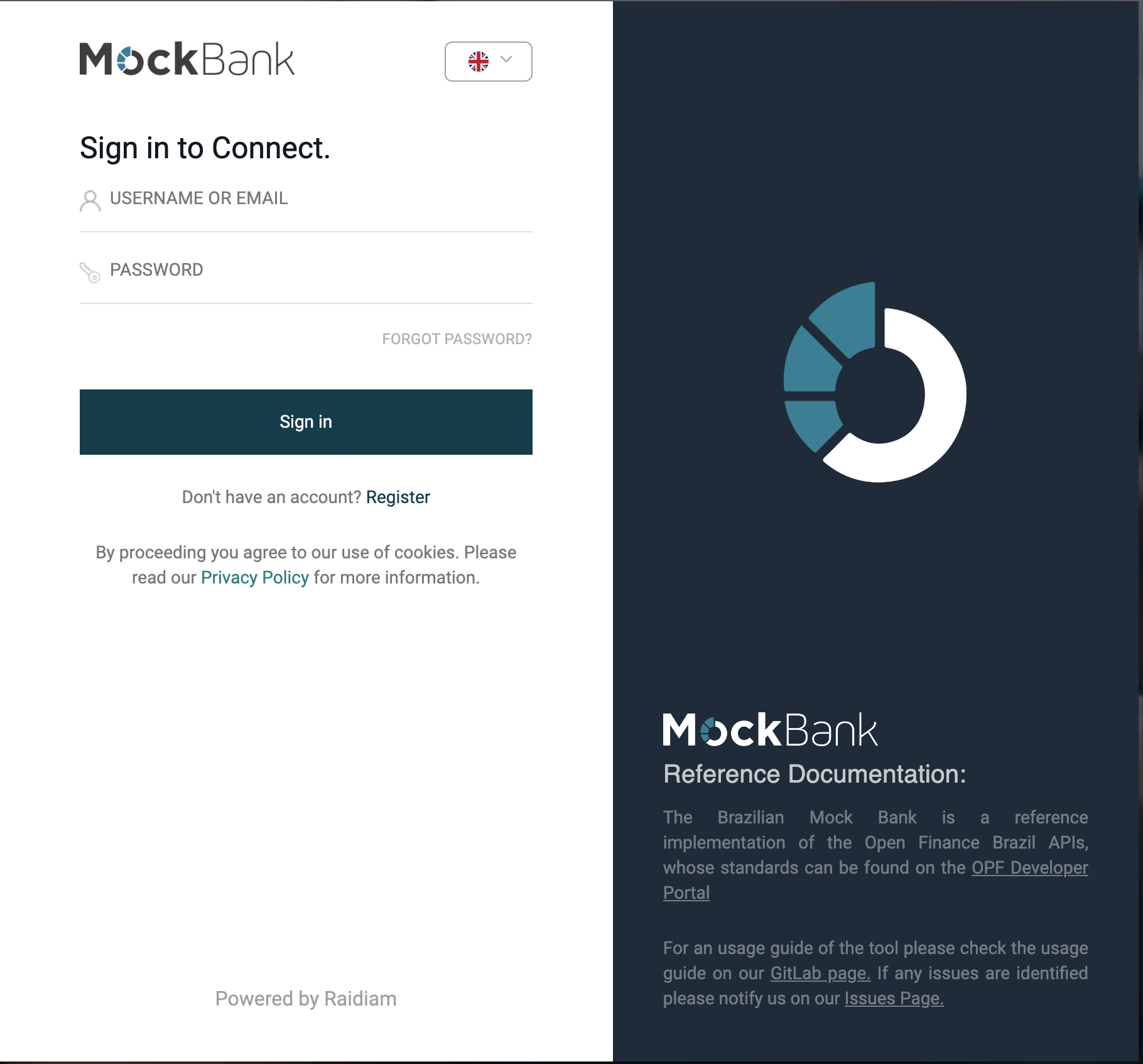
Use user and password to login into Mock bank and it will redirect to the Success or Error Page depending on the result of the Payment.
Updated 3 months ago